Mastering Sub Procedures in VB.NET: Your Ultimate Guide to Achieve Perfect Coding (VB.NET 2023)

Sub Procedures in VB.NET
Visual Basic .NET (VB.NET) is an object-oriented programming language used for developing desktop and web applications. One of the essential programming concepts in VB.NET is creating sub procedures. Sub procedures, also known as subroutines or methods, are reusable code blocks that perform a specific task. In this article, we will learn how to create sub procedures in VB.NET and use them in our programs.
Introduction
In programming, sub procedures are used to break down complex tasks into smaller, more manageable pieces of code. This modular approach makes the code more organized, easier to maintain, and less prone to errors. In VB.NET, sub procedures can be used to perform a wide range of tasks, such as manipulating data, displaying output, or processing user input.
What are Sub Procedures?
A sub procedure is a block of code that performs a specific task. It can be called from other parts of the program whenever needed. Sub procedures can be used to perform calculations, manipulate data, or carry out any other tasks that the program requires. Sub procedures can also be used to create custom functions, which can return a value to the calling code.
Sub Procedure vs Function in vb.net
In VB.NET, both sub procedures and functions are used to group together blocks of code that perform a specific task. However, there is a key difference between the two: sub procedures do not return a value, while functions do.
A sub procedure is a block of code that performs a specific task, but does not return a value. Sub procedures are often used to perform tasks that don’t require a return value, such as printing a message to the console, updating a database, or performing a calculation.
Here is an example of a sub procedure:
Sub PrintMessage(message As String) Console.WriteLine(message) End Sub
This sub procedure takes a string parameter called “message”, and prints it to the console. However, it does not return a value.
A function, on the other hand, is a block of code that performs a specific task and returns a value. Functions are often used to perform calculations or transformations on data, and return the result to the calling code.
Here is an example of a function:
Function CalculateSquareRoot(number As Double) As Double Dim result As Double = Math.Sqrt(number) Return result End Function
This function takes a double parameter called “number”, calculates its square root using the Math.Sqrt method, and returns the result.
To summarize, sub procedures are used to perform tasks that do not require a return value, while functions are used to perform calculations or transformations and return a value to the calling code. When writing code in VB.NET, it is important to choose the appropriate type of procedure based on the specific task at hand.
Syntax of Sub Procedures in VB.NET
The syntax of a sub procedure in VB.NET is as follows:
AccessModifier Sub ProcedureName (ParameterList) ' Code Block End Sub
The access modifier is optional and determines the visibility of the sub procedure. It can be Public, Private, Protected, Friend, or Protected Friend. The procedure name is the name of the sub procedure, and the parameter list is a comma-separated list of parameters that the sub procedure accepts. The code block contains the actual code that performs the task.
Example of Sub Procedures
Let’s look at an example of a sub procedure that adds two numbers and displays the result.
Public Sub AddNumbers(ByVal num1 As Integer, ByVal num2 As Integer) Dim result As Integer result = num1 + num2 Console.WriteLine("The result is: " & result) End Sub
In this example, the access modifier is Public, which means that the sub procedure can be called from any part of the program. The procedure name is AddNumbers, and it accepts two parameters, num1 and num2, both of which are integers. The code block performs the addition operation and displays the result using the Console.WriteLine method.
Some more complicated examples of sub procedures in VB.NET:
Here are some more complicated examples of sub procedures in VB.NET:
Example 1: Sorting an Array
Suppose we have an array of integers and we want to sort it in ascending order. We can create a sub procedure that takes the array as a parameter and sorts it using the bubble sort algorithm:
Public Sub BubbleSort(ByRef arr() As Integer) Dim n As Integer = arr.Length For i As Integer = 0 To n - 1 For j As Integer = 0 To n - i - 2 If arr(j) > arr(j + 1) Then Dim temp As Integer = arr(j) arr(j) = arr(j + 1) arr(j + 1) = temp End If Next Next End Sub
In this example, the BubbleSort sub procedure accepts an array of integers as a parameter. The procedure initializes a variable n with the length of the array and uses nested for loops to compare adjacent elements and swap them if necessary. The procedure uses the ByRef keyword to pass the array by reference, so any changes made to the array inside the procedure affect the original array.
Example 2: Drawing Shapes
Suppose we want to create a program that allows the user to draw shapes on the screen. We can create a sub procedure that takes a graphics object as a parameter and draws a shape using the methods of the graphics object:
Public Sub DrawRectangle(ByVal g As Graphics, ByVal x As Integer, ByVal y As Integer, ByVal width As Integer, ByVal height As Integer) Dim pen As New Pen(Color.Black) g.DrawRectangle(pen, x, y, width, height) End Sub
In this example, the DrawRectangle sub procedure accepts a graphics object as a parameter, along with the x and y coordinates, width, and height of the rectangle to be drawn. The procedure creates a pen object and uses the DrawRectangle method of the graphics object to draw the rectangle.
Example 3: File I/O
Suppose we want to create a program that reads data from a file and performs some calculations on it. We can create a sub procedure that takes a file name as a parameter and reads the data from the file:
Public Sub ReadFile(ByVal filename As String) Dim reader As New StreamReader(filename) Dim line As String While Not reader.EndOfStream line = reader.ReadLine() ' Process the line of data here... End While reader.Close() End Sub
In this example, the ReadFile sub procedure accepts a file name as a parameter and creates a StreamReader object to read data from the file. The procedure reads each line of the file using a While loop and processes the data as necessary. Finally, the procedure closes the StreamReader object.
These are just a few examples of the many ways sub procedures can be used in VB.NET programming. With sub procedures, we can create reusable blocks of code that make our programs more efficient, modular, and easier to maintain.
Passing Parameters to Sub Procedures
Sub procedures can accept parameters that are used as inputs for the code block. Parameters can be passed by value or by reference.
Passing by value means that a copy of the parameter’s value is passed to the sub procedure. This means that any changes made to the parameter inside the sub procedure do not affect the original value outside the sub procedure.
Passing by reference means that a reference to the original parameter is passed to the sub procedure. This means that any changes made to the parameter inside the sub procedure also affect the original value outside the sub procedure.
Here’s an example of passing parameters by value:
Public Sub MultiplyNumbers(ByVal num1 As Integer, ByVal num2 As Integer) Dim result As Integer result = num1 * num2 Console.WriteLine("The result is: " & result) End Sub Dim x As Integer = 5 Dim y As Integer = 10 MultiplyNumbers(x, y)
In this example, the MultiplyNumbers sub procedure accepts two parameters, num1 and num2, and multiplies them to get the result. The variables x and y are initialized with the values 5 and 10, respectively. The sub procedure is then called with these two variables as arguments. Since the parameters are passed by value, any changes made to the variables inside the sub procedure do not affect their values outside the sub procedure.
Here’s an example of passing parameters by reference:
Public Sub SwapNumbers(ByRef num1 As Integer, ByRef num2 As Integer) Dim temp As Integer temp = num1 num1 = num2 num2 = temp End Sub Dim x As Integer = 5 Dim y As Integer = 10 SwapNumbers(x, y) Console.WriteLine("x = " & x & ", y = " & y)
In this example, the SwapNumbers sub procedure accepts two parameters, num1 and num2, by reference. The sub procedure swaps the values of the two parameters using a temporary variable. The variables x and y are initialized with the values 5 and 10, respectively. The sub procedure is then called with these two variables as arguments. Since the parameters are passed by reference, the values of the variables are swapped inside the sub procedure, and their values outside the sub procedure are also changed.
Some more complicated examples of passing parameters to sub procedures in VB.NET:
Here are some more complicated examples of passing parameters to sub procedures in VB.NET:
Example 1: Calculating the Area of a Circle
Suppose we want to create a sub procedure that calculates the area of a circle given its radius. We can pass the radius as a parameter to the sub procedure and use it to calculate the area:
Public Sub CalculateCircleArea(ByVal radius As Double, ByVal lstOutput As ListBox) Dim area As Double = Math.PI * radius * radius lstOutput.Items.Add(String.Format("The area of the circle with radius {0} is {1}.", radius, area)) End Sub
Here, we have added an additional parameter called listbox1, which represents the listbox1 control where the output should be displayed. we use listbox1.Items.Add to add the output as a new item to the listbox control.
To call this sub procedure and display the output in a listbox, you would need to pass the appropriate arguments. For example, you might have a button on your form that calculates the area of a circle based on the input radius and displays the result in a listbox:
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click ' Get the radius value from a textbox control Dim radius As Double = CDbl(15) ' Call the CalculateCircleArea sub procedure, passing the radius and the listbox control CalculateCircleArea(radius, ListBox1) End Sub
Here, we first retrieve the radius value which is 15 in this case. We then call the modified CalculateCircleArea sub procedure, passing the radius value and the listbox control (Listbox1). The sub procedure will calculate the area of the circle and add a new item to the listbox control with the formatted output string as shown below.
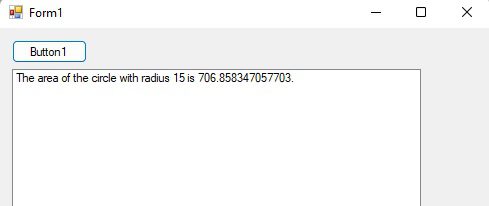
Example 2: Searching for a Value in an Array
Suppose we have an array of integers and we want to create a sub procedure that searches for a particular value in the array. We can pass the array and the value to search for as parameters to the sub procedure and use them to search the array:
Public Sub SearchArray(ByVal arr() As Integer, ByVal value As Integer, ByVal lstOutput As ListBox) For i As Integer = 0 To arr.Length - 1 If arr(i) = value Then lstOutput.Items.Add(String.Format("The value {0} was found at index {1}.", value, i)) Exit Sub End If Next lstOutput.Items.Add(String.Format("The value {0} was not found in the array.", value)) End Sub
Here, we have added an additional parameter called lstOutput, which represents the listbox control where the output should be displayed. we use lstOutput.Items.Add to add the output as a new item to the listbox control.
To call this sub procedure and display the output in a listbox, you would need to pass the appropriate arguments. For example, you might have a button on your form that searches an array of integers for a particular value and displays the result in a listbox:
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click Dim arr() As Integer = {5, 3, 8, 2, 9} ' Get the value to search for from a textbox control Dim value As Integer = CInt(8) ' Call the SearchArray sub procedure, passing the array, the value, and the listbox control SearchArray(arr, value, ListBox1 ) End Sub
Here, we first create an array of integers and initialize it with some values. We then retrieve the value to search for i.e 8 (You should be clear here that indexing will always be started at 0). Finally, we call the SearchArray sub procedure, passing the array, the value, and the listbox control (Listbox1). The sub procedure will search the array for the specified value and add a new item to the listbox control with the formatted output string as shown below.

Example 3: Sorting an Array with Custom Comparer
Suppose we have an array of strings and we want to sort it using a custom comparison function. We can pass the array and the comparison function as parameters to the sub procedure and use them to sort the array:
Public Sub SortArrayWithComparer(ByVal arr() As String, ByVal comparer As Comparison(Of String), ByVal listBox As ListBox) Array.Sort(arr, comparer) For Each item As String In arr listBox.Items.Add(item) Next End Sub
In this method, we have added a third parameter listBox of type ListBox. This parameter will be used to pass the reference of the ListBox control on which we want to display the sorted array items.
we are now using a For Each loop to iterate over each item in the sorted array and adding it to the Items collection of the listBox control using the Add method.
To call this method and display the output in a ListBox, you can do something like this:
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click Dim arr() As String = {"cat", "dog", "elephant", "ant", "zebra"} ListBox1.Items.Clear() SortArrayWithComparer(arr, Function(x, y) x.CompareTo(y), ListBox1) End Sub
Here, we first declare an array of strings and then clear the items of the ListBox1 control. We then call the SortArrayWithComparer method and pass the array, a comparison function, and the reference of ListBox1 control as parameters. The comparison function here is Function(x, y) x.CompareTo(y) which compares two strings based on their natural order.
The output is shown below:

Calling Sub Procedures
To call a sub procedure, we simply use its name followed by any required arguments in parentheses. Here’s an example:
Public Sub Main() Dim x As Integer = 5 Dim y As Integer = 10 AddNumbers(x, y) End Sub
In this example, the Main sub procedure initializes two variables, x and y, with the values 5 and 10, respectively. The AddNumbers sub procedure is then called with these two variables as arguments.
Some more complicated examples of calling sub procedures in VB.NET:
Here are some more complicated examples of calling sub procedures in VB.NET:
Example 1: Calling a Sub Procedure with Multiple Parameters
Suppose we have a sub procedure that calculates the area of a rectangle given its length and width.
Public Sub CalculateRectangleArea(ByVal length As Double, ByVal width As Double) Dim area As Double = 0.0 area = length * width ListBox1.Items.Add("The area of your provided parameters is = " & area) End Sub
We can call this sub procedure by passing the length and width as parameters:
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click Dim length As Double = 5 Dim width As Double = 3 CalculateRectangleArea(length, width) End Sub
Run the app and click the button to see the following output:

Example 2: Calling a Sub Procedure with a Return Value
Suppose we have a sub procedure that calculates the square root of a number and returns the result. We can call this sub procedure and assign its return value to a variable:
Public Function CalculateSquareRoot(ByVal number As Double) As Double Dim result As Double = Math.Sqrt(number) Return result End Function
In this method, we are using the Math.Sqrt method to calculate the square root of the input number and returning the result using the Return keyword.
To display the output in a ListBox instead of the console, you can modify the calling code like this:
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click Dim number As Double = 16 ListBox1.Items.Clear() Dim result As Double = CalculateSquareRoot(number) ListBox1.Items.Add(String.Format("The square root of {0} is {1}.", number, result)) End Sub
Here, we first clear the items of the ListBox1 control. We then call the CalculateSquareRoot function to calculate the square root of the number variable and store the result in the result variable. Finally, we add the result to the Items collection of the ListBox1 control using the Add method along with a formatted string that displays the input number and the calculated square root.
Run the app and click the button to see the following output:
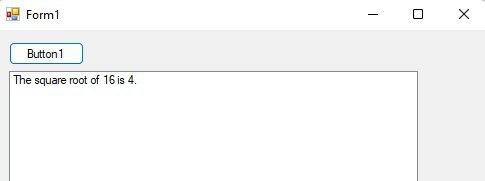
Example 3: Calling a Sub Procedure with Optional Parameters
Suppose we have a sub procedure that takes two parameters, but one of them is optional. We can call this sub procedure with both parameters, or with just the required parameter:
Public Sub PrintMessage(ByVal requiredParam As String, Optional ByVal optionalParam As String = "Optional parameter") Console.WriteLine(requiredParam + " " + optionalParam) End Sub
In this method, we have a required parameter requiredParam which is of type String and an optional parameter optionalParam which is also of type String. If the optionalParam is not provided, it defaults to the value “Optional parameter”.
To display the results in a ListBox, you can call code like this:
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click Dim requiredParam As String = "Hello" Dim optionalParam As String = "World" ListBox1.Items.Clear() PrintMessage(requiredParam) PrintMessage(requiredParam, optionalParam) ListBox1.Items.Add(requiredParam + " " + "Optional parameter") ListBox1.Items.Add(requiredParam + " " + optionalParam) End Sub
Here, we first clear the items of the ListBox1 control. We then call the PrintMessage method twice, once with only the requiredParam argument, and once with both requiredParam and optionalParam arguments. We then add the resulting strings to the Items collection of the ListBox1 control using the Add method.
The output is as:

Conclusion
In this article, we have learned how to create sub procedures in VB.NET and use them in our programs. Sub procedures are essential programming constructs that make our code more modular, organized, and easier to maintain. We have also learned how to pass parameters to sub procedures, call sub procedures, and use them in our programs.
Sub procedures play a significant role in VB.NET programming as they provide a structured and organized approach to coding. They allow developers to break down complex tasks into smaller, manageable components that can be reused and called upon when necessary. This saves time and effort in the long run and makes the code easier to maintain and update.
In addition to creating sub procedures, we have also discussed how to pass parameters to sub procedures, including by reference and by value. This allows us to customize the behavior of the sub procedure based on the specific requirements of the program.
Furthermore, we have explored how to call sub procedures and have looked at some more complicated examples of sub procedures, including sorting arrays, searching arrays, and calculating square roots. We have also seen how sub procedures differ from functions and when to use each.
Sub procedures are a fundamental building block of VB.NET programming, and mastering them is essential for any developer looking to write efficient and maintainable code. By using sub procedures in our programs, we can improve the overall structure and organization of our code, making it easier to read, update, and maintain.
In this article, we discussed how to create and use sub procedures in VB.NET. Sub procedures are a type of procedure that do not return a value and are used to execute a set of instructions. We learned how to pass parameters to sub procedures, how to call sub procedures, and how to use them in our programs. We also explored some examples of sub procedures, including how to calculate the area of a circle, search an array for a value, and sort an array using a comparer. Finally, we compared sub procedures to functions and discussed the differences between the two.
By using sub procedures in our code, we can improve its modularity and organization, making it easier to maintain and update. The examples provided in this article demonstrate how sub procedures can be used in a variety of scenarios, from simple mathematical calculations to more complex data processing tasks. By understanding how to create and use sub procedures in VB.NET, developers can enhance the functionality and efficiency of their programs.
Overall, sub procedures are an important component of VB.NET programming, and understanding how to use them effectively is a valuable skill for developers. By following the examples and guidelines outlined in this article, developers can create more efficient, maintainable, and flexible code using sub procedures.
Q: 1. What is the difference between a sub procedure and a function in VB.NET?
A: A sub procedure is a block of code that performs a specific task, whereas a function is a sub procedure that returns a value to the calling code.
Q: 2. Can a sub procedure have multiple parameters in VB.NET?
A: Yes, a sub procedure can have multiple parameters in VB.NET.
Q: 3. What is the difference between passing parameters by value and by reference in VB.NET?
A: Passing by value means that a copy of the parameter’s value is passed to the sub procedure, while passing by reference means that a reference to the original parameter is passed to the sub procedure.
Q: 4. How can I call a sub procedure from another sub procedure in VB.NET?
A: To call a sub procedure from another sub procedure, simply use its name followed by any required arguments in parentheses.
Q: 5. Can I use sub procedures in web applications developed in VB.NET?
A: Yes, sub procedures can be used in both desktop and web applications developed in VB.NET.
Q: 6. What is the purpose of using the ByVal and ByRef keywords in sub procedures?
A: The ByVal keyword is used to pass a value type parameter by value, while the ByRef keyword is used to pass a value type parameter by reference. ByVal creates a copy of the parameter value, while ByRef allows the sub procedure to modify the original parameter value.
Q: 7. Can a sub procedure have a return type?
A: No, a sub procedure does not have a return type. It only performs a set of operations and may modify the values of its parameters.
Q: 8. Can sub procedures be called from other sub procedures?
A: Yes, sub procedures can be called from other sub procedures as long as they are declared within the same module or class.
More Links